While working through the tutorial for SPFx @ Microsoft Docs, I came across a section that discusses how to get data from the Graph API. This was just a call to Graph to get messages from “/me/mail”. The call discussed would fetch the last 5 email messages from the currently logged in user’s inbox. Here is the actual code:
this.context.msGraphClientFactory .getClient() .then((client: MSGraphClient): void => { client .api('/me/messages') .top(5) .orderby("receivedDateTime desc") .get((error, messages: any, rawResponse?: any) => { this.domElement.innerHTML = ` <div class="${ styles.myFirstGraphWebPart}"> <div class="${ styles.container}"> <div class="${ styles.row}"> <div class="${ styles.column}"> <span class="${ styles.title}">Welcome to SharePoint!</span> <p class="${ styles.subTitle}">Using Microsoft Graph API in SharePoint Framework.</p> <div id="spListContainer" /> </div> </div> </div> </div>`; this._renderEmailList(messages.value); }); });
Now, there’s a few things happening here. The obvious one is that dot notation. This is called “method chaining” and it gets some getting used to. The next thing is “then”. You see how the first call is to the Microsoft Graph Client Factory, to get a Graph Client, and the next method is called inside a “then()”. This is the first nudge towards asynchronous functions. Wait, you say, what’s happening inside that “then()”? That ((client: MSGraphClient): void => {…}) is new!? Well, that’s where we find the next two things to discuss: anonymous functions and the fat arrow notation.
Let’s get started.
Method chaining / Dot notation
Typescript (and indeed JavaScript) support method chaining. This is a way to make nested methods more readable. Why? Because, if you think about it, nested methods are executed the opposite way to the way they are written. It’s not the worst (and, if you’re like me, you’re probably so very used to it that you don’t even think about it). It is however less readable than having your code execute the exact way it is written. Think about the following code:
SayHello(GrabName(InitialiseNewPersonForm()));
This is fine, right? What’s happening here? You call the SayHello method (that needs a name to say hello to, so it calls the GrabName method, (that needs a form to get the name from so it calls the InitialiseNewPersonForm())). And how are all those executed? The exact opposite way to which the are being called. First, the form will be initialised, then the name will be extracted from the form, then the code will say hello to that name.
Method chaining could turn the above into something like the following:
InitialiseNewPersonForm().GrabName().SayHello();
(this is an oversimplification and does not take into account that values must be returned, but we’ll examine this later in the post)
This is much easier to read and understand, isn’t it? That’s method chaining. Everything following something is building on what was written before it.
Async/Await/Then
Going back to the Microsoft Graph Client snippet above, what is that “then” doing there?
this.context.msGraphClientFactory .getClient() .then((client: MSGraphClient): void => {…}
Lexically speaking, “then” is pretty self-explanatory. Do something, THEN (once you’re done with that step), do something else. But, what does it really mean in the context of the code above (or any code). The thing to understand is… Promises (as Megadeth would sing).
Asynchronous functions make a Promise. In case they keep their promise, the “then” part of the function is executed. There’s always a possibility that the promise will be broken. In that case, the catch part of the function is executed. Which brings us to the states of a Promise.
A Promise can be in one of three states:
- Pending: this is the initial state. A Promise is made and it’s neither fulfilled, nor rejected.
- Fulfilled: the happy state. A Promise was successful and the promised object is returned.
- Rejected: the sad state. A Promise was broken and the reason is returned in the form of an Error.
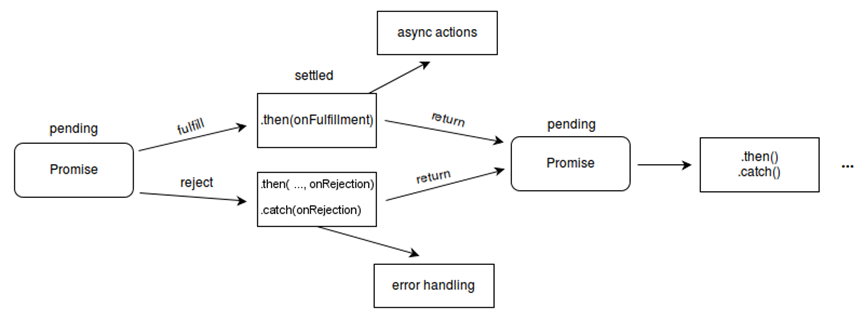
A diagram illustrating the states of a Promise in TypeScript / JavaScript
You would think, based on the above, that the Promise prototype only has 2 methods .then() for success and .catch() for failure. There is in fact a third method .finally() which creates a Promise to be resolved after the initial promise is settled, be it a fulfilled or rejected.
Fat Arrow =>
In the code snippet above, the anonymous function definition comes first, but there’s no way to properly discuss it without mentioning the fat arrow. In simple words, the fat arrow => means that whatever follows is what the anonymous function before it needs to do. Jump below to better understand.
Anonymous Functions
You’re probably aware of function definition. You give it a name, you type it, you set an access modifier, etc. Once you’re done and you want to use it, you call it in your code by name.
Anonymous functions allow you to define your function and execute it in place.
Would it be easier to understand with an example? Consider a method that adds two numbers, like this:
private function add(x: number, y: number):Number { return x+y; }
This makes sense, right? In order to use it, you call the function by name and give it the required parameters, like so: add(2, 3);
So, how would this look as an anonymous function? Like so:
(x:number, y:number):Number => { return x+y; }
Mind you, ECMAScript 6 is what allows the shorthand notation that means you can completely omit the “function” keyword. These are called arrow functions .
References:
- Make a chain of methods with TypeScript
- Understanding Method Chaining In Javascript
- MDN – Standard built-in JavaScript Objects: Promise
- Understand promises before you start using async/await
- 6 Reasons Why JavaScript Async/Await Blows Promises Away (Tutorial)
- Keep Your Promises in TypeScript using async/await
- JavaScript Promises and Async/Await: As Fast As Possible
- TypeScript – Arrow Function
- Comparison Between ECMAScript 5 And ECMAScript 6 Versions Of JavaScript
- Functions
- Introduction to TypeScript Functions: Anonymous Functions and More
- Typescript anonymous functions
- TypeScript Anonymous Functions – Syntax & Examples